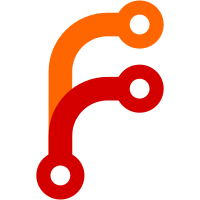
1) Removed virtual methods. There was not really need for them. 2) Some of the virtual methods were using conversion to Lines, which was unnecessary and expensive. 3) Removed some nearest element search methods from Point.
130 lines
4.1 KiB
Plaintext
130 lines
4.1 KiB
Plaintext
%module{Slic3r::XS};
|
|
|
|
%{
|
|
#include <xsinit.h>
|
|
#include "libslic3r/Point.hpp"
|
|
#include "libslic3r/Line.hpp"
|
|
#include "libslic3r/Polygon.hpp"
|
|
#include "libslic3r/Polyline.hpp"
|
|
%}
|
|
|
|
%name{Slic3r::Point} class Point {
|
|
Point(int _x = 0, int _y = 0);
|
|
~Point();
|
|
Clone<Point> clone()
|
|
%code{% RETVAL=THIS; %};
|
|
void scale(double factor)
|
|
%code{% *THIS *= factor; %};
|
|
void translate(double x, double y)
|
|
%code{% *THIS += Point(x, y); %};
|
|
SV* arrayref()
|
|
%code{% RETVAL = to_SV_pureperl(THIS); %};
|
|
SV* pp()
|
|
%code{% RETVAL = to_SV_pureperl(THIS); %};
|
|
int x()
|
|
%code{% RETVAL = (*THIS)(0); %};
|
|
int y()
|
|
%code{% RETVAL = (*THIS)(1); %};
|
|
void set_x(int val)
|
|
%code{% (*THIS)(0) = val; %};
|
|
void set_y(int val)
|
|
%code{% (*THIS)(1) = val; %};
|
|
Clone<Point> nearest_point(Points points)
|
|
%code{% RETVAL = nearest_point(points, *THIS).first; %};
|
|
double distance_to(Point* point)
|
|
%code{% RETVAL = (*point - *THIS).cast<double>().norm(); %};
|
|
double distance_to_line(Line* line)
|
|
%code{% RETVAL = line->distance_to(*THIS); %};
|
|
double perp_distance_to_line(Line* line)
|
|
%code{% RETVAL = line->perp_distance_to(*THIS); %};
|
|
double ccw(Point* p1, Point* p2)
|
|
%code{% RETVAL = cross2((*p1 - *THIS).cast<double>(), (*p2 - *p1).cast<double>()); %};
|
|
Point* negative()
|
|
%code{% RETVAL = new Point(- *THIS); %};
|
|
std::string serialize() %code{% char buf[2048]; sprintf(buf, "%ld,%ld", (*THIS)(0), (*THIS)(1)); RETVAL = buf; %};
|
|
|
|
%{
|
|
|
|
void
|
|
Point::rotate(angle, center_sv)
|
|
double angle;
|
|
SV* center_sv;
|
|
CODE:
|
|
Point center;
|
|
from_SV_check(center_sv, ¢er);
|
|
THIS->rotate(angle, center);
|
|
|
|
bool
|
|
Point::coincides_with(point_sv)
|
|
SV* point_sv;
|
|
CODE:
|
|
Point point;
|
|
from_SV_check(point_sv, &point);
|
|
RETVAL = (*THIS) == point;
|
|
OUTPUT:
|
|
RETVAL
|
|
|
|
%}
|
|
|
|
};
|
|
|
|
%name{Slic3r::Pointf} class Vec2d {
|
|
Vec2d(double _x = 0, double _y = 0);
|
|
~Vec2d();
|
|
Clone<Vec2d> clone()
|
|
%code{% RETVAL = THIS; %};
|
|
SV* arrayref()
|
|
%code{% RETVAL = to_SV_pureperl(THIS); %};
|
|
SV* pp()
|
|
%code{% RETVAL = to_SV_pureperl(THIS); %};
|
|
double x()
|
|
%code{% RETVAL = (*THIS)(0); %};
|
|
double y()
|
|
%code{% RETVAL = (*THIS)(1); %};
|
|
void set_x(double val)
|
|
%code{% (*THIS)(0) = val; %};
|
|
void set_y(double val)
|
|
%code{% (*THIS)(1) = val; %};
|
|
void translate(double x, double y)
|
|
%code{% *THIS += Vec2d(x, y); %};
|
|
void scale(double factor)
|
|
%code{% *THIS *= factor; %};
|
|
void rotate(double angle, Vec2d* center)
|
|
%code{% *THIS = Eigen::Translation2d(*center) * Eigen::Rotation2Dd(angle) * Eigen::Translation2d(- *center) * Eigen::Vector2d((*THIS)(0), (*THIS)(1)); %};
|
|
Vec2d* negative()
|
|
%code{% RETVAL = new Vec2d(- *THIS); %};
|
|
Vec2d* vector_to(Vec2d* point)
|
|
%code{% RETVAL = new Vec2d(*point - *THIS); %};
|
|
std::string serialize() %code{% char buf[2048]; sprintf(buf, "%lf,%lf", (*THIS)(0), (*THIS)(1)); RETVAL = buf; %};
|
|
};
|
|
|
|
%name{Slic3r::Pointf3} class Vec3d {
|
|
Vec3d(double _x = 0, double _y = 0, double _z = 0);
|
|
~Vec3d();
|
|
Clone<Vec3d> clone()
|
|
%code{% RETVAL = THIS; %};
|
|
double x()
|
|
%code{% RETVAL = (*THIS)(0); %};
|
|
double y()
|
|
%code{% RETVAL = (*THIS)(1); %};
|
|
double z()
|
|
%code{% RETVAL = (*THIS)(2); %};
|
|
void set_x(double val)
|
|
%code{% (*THIS)(0) = val; %};
|
|
void set_y(double val)
|
|
%code{% (*THIS)(1) = val; %};
|
|
void set_z(double val)
|
|
%code{% (*THIS)(2) = val; %};
|
|
void translate(double x, double y, double z)
|
|
%code{% *THIS += Vec3d(x, y, z); %};
|
|
void scale(double factor)
|
|
%code{% *THIS *= factor; %};
|
|
double distance_to(Vec3d* point)
|
|
%code{% RETVAL = (*point - *THIS).norm(); %};
|
|
Vec3d* negative()
|
|
%code{% RETVAL = new Vec3d(- *THIS); %};
|
|
Vec3d* vector_to(Vec3d* point)
|
|
%code{% RETVAL = new Vec3d(*point - *THIS); %};
|
|
std::string serialize() %code{% char buf[2048]; sprintf(buf, "%lf,%lf,%lf", (*THIS)(0), (*THIS)(1), (*THIS)(2)); RETVAL = buf; %};
|
|
};
|