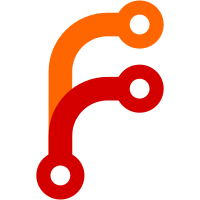
DynamicPrintConfig::full_print_config() new cheaper constructors of DynamicConfig / DynamicPrintConfig from ConfigBase Unit tests: ported test_model from upstream Slic3r, thanks @lordofhyphens Unit tests refactored to use less autos and initializer lists for readibility, DynamicPrintConfig is handled by value, not by shared pointer.
236 lines
12 KiB
Text
236 lines
12 KiB
Text
%module{Slic3r::XS};
|
|
|
|
%{
|
|
#include <xsinit.h>
|
|
#include "libslic3r/PrintConfig.hpp"
|
|
%}
|
|
|
|
%name{Slic3r::Config} class DynamicPrintConfig {
|
|
DynamicPrintConfig();
|
|
~DynamicPrintConfig();
|
|
static DynamicPrintConfig* new_from_defaults();
|
|
DynamicPrintConfig* DynamicPrintConfig::new_from_defaults()
|
|
%code{% RETVAL = DynamicPrintConfig::new_from_defaults_keys(FullPrintConfig::defaults().keys()); %};
|
|
static DynamicPrintConfig* new_from_defaults_keys(std::vector<std::string> keys);
|
|
DynamicPrintConfig* clone() %code{% RETVAL = new DynamicPrintConfig(*THIS); %};
|
|
DynamicPrintConfig* clone_only(std::vector<std::string> keys)
|
|
%code{% RETVAL = new DynamicPrintConfig(); RETVAL->apply_only(*THIS, keys, true); %};
|
|
bool has(t_config_option_key opt_key);
|
|
SV* as_hash()
|
|
%code{% RETVAL = ConfigBase__as_hash(THIS); %};
|
|
SV* get(t_config_option_key opt_key)
|
|
%code{% RETVAL = ConfigBase__get(THIS, opt_key); %};
|
|
SV* get_at(t_config_option_key opt_key, int i)
|
|
%code{% RETVAL = ConfigBase__get_at(THIS, opt_key, i); %};
|
|
SV* get_value(t_config_option_key opt_key)
|
|
%code{%
|
|
const ConfigOptionDef *def = THIS->def()->get(opt_key);
|
|
RETVAL = (def != nullptr && ! def->ratio_over.empty()) ?
|
|
newSVnv(THIS->get_abs_value(opt_key)) :
|
|
ConfigBase__get(THIS, opt_key);
|
|
%};
|
|
bool set(t_config_option_key opt_key, SV* value)
|
|
%code{% RETVAL = ConfigBase__set(THIS, opt_key, value); %};
|
|
bool set_deserialize(t_config_option_key opt_key, SV* str)
|
|
%code{% RETVAL = ConfigBase__set_deserialize(THIS, opt_key, str); %};
|
|
void set_ifndef(t_config_option_key opt_key, SV* value, bool deserialize = false)
|
|
%code{% ConfigBase__set_ifndef(THIS, opt_key, value, deserialize); %};
|
|
std::string opt_serialize(t_config_option_key opt_key);
|
|
double get_abs_value(t_config_option_key opt_key);
|
|
%name{get_abs_value_over}
|
|
double get_abs_value(t_config_option_key opt_key, double ratio_over);
|
|
void apply(DynamicPrintConfig* other)
|
|
%code{% THIS->apply(*other, true); %};
|
|
std::vector<std::string> diff(DynamicPrintConfig* other)
|
|
%code{% RETVAL = THIS->diff(*other); %};
|
|
bool equals(DynamicPrintConfig* other)
|
|
%code{% RETVAL = THIS->equals(*other); %};
|
|
void apply_static(StaticPrintConfig* other)
|
|
%code{% THIS->apply(*other, true); %};
|
|
%name{get_keys} std::vector<std::string> keys();
|
|
void erase(t_config_option_key opt_key);
|
|
void normalize();
|
|
%name{setenv} void setenv_();
|
|
double min_object_distance() %code{% PrintConfig cfg; cfg.apply(*THIS, true); RETVAL = cfg.min_object_distance(); %};
|
|
static DynamicPrintConfig* load(char *path)
|
|
%code%{
|
|
auto config = new DynamicPrintConfig();
|
|
try {
|
|
config->load(path);
|
|
RETVAL = config;
|
|
} catch (std::exception& e) {
|
|
delete config;
|
|
croak("Error extracting configuration from %s:\n%s\n", path, e.what());
|
|
}
|
|
%};
|
|
void save(std::string file);
|
|
int validate() %code%{
|
|
std::string err = THIS->validate();
|
|
if (! err.empty())
|
|
croak("Configuration is not valid: %s\n", err.c_str());
|
|
RETVAL = 1;
|
|
%};
|
|
};
|
|
|
|
%name{Slic3r::Config::Static} class StaticPrintConfig {
|
|
static StaticPrintConfig* new_GCodeConfig()
|
|
%code{% RETVAL = new GCodeConfig(); %};
|
|
static StaticPrintConfig* new_PrintConfig()
|
|
%code{% RETVAL = static_cast<GCodeConfig*>(new PrintConfig()); %};
|
|
static StaticPrintConfig* new_PrintObjectConfig()
|
|
%code{% RETVAL = new PrintObjectConfig(); %};
|
|
static StaticPrintConfig* new_PrintRegionConfig()
|
|
%code{% RETVAL = new PrintRegionConfig(); %};
|
|
static StaticPrintConfig* new_FullPrintConfig()
|
|
%code{% RETVAL = static_cast<GCodeConfig*>(new FullPrintConfig()); %};
|
|
~StaticPrintConfig();
|
|
bool has(t_config_option_key opt_key);
|
|
SV* as_hash()
|
|
%code{% RETVAL = ConfigBase__as_hash(THIS); %};
|
|
SV* get(t_config_option_key opt_key)
|
|
%code{% RETVAL = ConfigBase__get(THIS, opt_key); %};
|
|
SV* get_at(t_config_option_key opt_key, int i)
|
|
%code{% RETVAL = ConfigBase__get_at(THIS, opt_key, i); %};
|
|
bool set(t_config_option_key opt_key, SV* value)
|
|
%code{% RETVAL = StaticConfig__set(THIS, opt_key, value); %};
|
|
bool set_deserialize(t_config_option_key opt_key, SV* str)
|
|
%code{% RETVAL = ConfigBase__set_deserialize(THIS, opt_key, str); %};
|
|
void set_ifndef(t_config_option_key opt_key, SV* value, bool deserialize = false)
|
|
%code{% ConfigBase__set_ifndef(THIS, opt_key, value, deserialize); %};
|
|
std::string opt_serialize(t_config_option_key opt_key);
|
|
double get_abs_value(t_config_option_key opt_key);
|
|
%name{get_abs_value_over}
|
|
double get_abs_value(t_config_option_key opt_key, double ratio_over);
|
|
void apply_static(StaticPrintConfig* other)
|
|
%code{% THIS->apply(*other, true); %};
|
|
void apply_dynamic(DynamicPrintConfig* other)
|
|
%code{% THIS->apply(*other, true); %};
|
|
%name{get_keys} std::vector<std::string> keys();
|
|
std::string get_extrusion_axis()
|
|
%code{%
|
|
if (GCodeConfig* config = dynamic_cast<GCodeConfig*>(THIS)) {
|
|
RETVAL = config->get_extrusion_axis();
|
|
} else {
|
|
CONFESS("This StaticConfig object does not provide get_extrusion_axis()");
|
|
}
|
|
%};
|
|
%name{setenv} void setenv_();
|
|
double min_object_distance() %code{% RETVAL = PrintConfig::min_object_distance(THIS); %};
|
|
static StaticPrintConfig* load(char *path)
|
|
%code%{
|
|
auto config = new FullPrintConfig();
|
|
try {
|
|
config->load(path);
|
|
RETVAL = static_cast<GCodeConfig*>(config);
|
|
} catch (std::exception& e) {
|
|
delete config;
|
|
croak("Error extracting configuration from %s:\n%s\n", path, e.what());
|
|
}
|
|
%};
|
|
|
|
void save(std::string file);
|
|
};
|
|
|
|
%package{Slic3r::Config};
|
|
|
|
%{
|
|
PROTOTYPES: DISABLE
|
|
|
|
SV*
|
|
print_config_def()
|
|
CODE:
|
|
t_optiondef_map &def = *const_cast<t_optiondef_map*>(&Slic3r::print_config_def.options);
|
|
|
|
HV* options_hv = newHV();
|
|
for (t_optiondef_map::iterator oit = def.begin(); oit != def.end(); ++oit) {
|
|
HV* hv = newHV();
|
|
|
|
t_config_option_key opt_key = oit->first;
|
|
ConfigOptionDef* optdef = &oit->second;
|
|
|
|
const char* opt_type;
|
|
if (optdef->type == coFloat || optdef->type == coFloats || optdef->type == coFloatOrPercent) {
|
|
opt_type = "f";
|
|
} else if (optdef->type == coPercent || optdef->type == coPercents) {
|
|
opt_type = "percent";
|
|
} else if (optdef->type == coInt || optdef->type == coInts) {
|
|
opt_type = "i";
|
|
} else if (optdef->type == coString) {
|
|
opt_type = "s";
|
|
} else if (optdef->type == coStrings) {
|
|
opt_type = "s@";
|
|
} else if (optdef->type == coPoint || optdef->type == coPoints) {
|
|
opt_type = "point";
|
|
} else if (optdef->type == coPoint3) {
|
|
opt_type = "point3";
|
|
} else if (optdef->type == coBool || optdef->type == coBools) {
|
|
opt_type = "bool";
|
|
} else if (optdef->type == coEnum) {
|
|
opt_type = "select";
|
|
} else {
|
|
throw "Unknown option type";
|
|
}
|
|
(void)hv_stores( hv, "type", newSVpv(opt_type, 0) );
|
|
(void)hv_stores( hv, "gui_type", newSVpvn(optdef->gui_type.c_str(), optdef->gui_type.length()) );
|
|
(void)hv_stores( hv, "gui_flags", newSVpvn(optdef->gui_flags.c_str(), optdef->gui_flags.length()) );
|
|
(void)hv_stores( hv, "label", newSVpvn_utf8(optdef->label.c_str(), optdef->label.length(), true) );
|
|
if (!optdef->full_label.empty())
|
|
(void)hv_stores( hv, "full_label", newSVpvn_utf8(optdef->full_label.c_str(), optdef->full_label.length(), true) );
|
|
(void)hv_stores( hv, "category", newSVpvn_utf8(optdef->category.c_str(), optdef->category.length(), true) );
|
|
(void)hv_stores( hv, "tooltip", newSVpvn_utf8(optdef->tooltip.c_str(), optdef->tooltip.length(), true) );
|
|
(void)hv_stores( hv, "sidetext", newSVpvn_utf8(optdef->sidetext.c_str(), optdef->sidetext.length(), true) );
|
|
(void)hv_stores( hv, "cli", newSVpvn(optdef->cli.c_str(), optdef->cli.length()) );
|
|
(void)hv_stores( hv, "ratio_over", newSVpvn(optdef->ratio_over.c_str(), optdef->ratio_over.length()) );
|
|
(void)hv_stores( hv, "multiline", newSViv(optdef->multiline ? 1 : 0) );
|
|
(void)hv_stores( hv, "full_width", newSViv(optdef->full_width ? 1 : 0) );
|
|
(void)hv_stores( hv, "readonly", newSViv(optdef->readonly ? 1 : 0) );
|
|
(void)hv_stores( hv, "height", newSViv(optdef->height) );
|
|
(void)hv_stores( hv, "width", newSViv(optdef->width) );
|
|
(void)hv_stores( hv, "min", newSViv(optdef->min) );
|
|
(void)hv_stores( hv, "max", newSViv(optdef->max) );
|
|
|
|
// aliases
|
|
if (!optdef->aliases.empty()) {
|
|
AV* av = newAV();
|
|
av_fill(av, optdef->aliases.size()-1);
|
|
for (std::vector<t_config_option_key>::iterator it = optdef->aliases.begin(); it != optdef->aliases.end(); ++it)
|
|
av_store(av, it - optdef->aliases.begin(), newSVpvn(it->c_str(), it->length()));
|
|
(void)hv_stores( hv, "aliases", newRV_noinc((SV*)av) );
|
|
}
|
|
|
|
// shortcut
|
|
if (!optdef->shortcut.empty()) {
|
|
AV* av = newAV();
|
|
av_fill(av, optdef->shortcut.size()-1);
|
|
for (std::vector<t_config_option_key>::iterator it = optdef->shortcut.begin(); it != optdef->shortcut.end(); ++it)
|
|
av_store(av, it - optdef->shortcut.begin(), newSVpvn(it->c_str(), it->length()));
|
|
(void)hv_stores( hv, "shortcut", newRV_noinc((SV*)av) );
|
|
}
|
|
|
|
// enum_values
|
|
if (!optdef->enum_values.empty()) {
|
|
AV* av = newAV();
|
|
av_fill(av, optdef->enum_values.size()-1);
|
|
for (std::vector<std::string>::iterator it = optdef->enum_values.begin(); it != optdef->enum_values.end(); ++it)
|
|
av_store(av, it - optdef->enum_values.begin(), newSVpvn(it->c_str(), it->length()));
|
|
(void)hv_stores( hv, "values", newRV_noinc((SV*)av) );
|
|
}
|
|
|
|
// enum_labels
|
|
if (!optdef->enum_labels.empty()) {
|
|
AV* av = newAV();
|
|
av_fill(av, optdef->enum_labels.size()-1);
|
|
for (std::vector<std::string>::iterator it = optdef->enum_labels.begin(); it != optdef->enum_labels.end(); ++it)
|
|
av_store(av, it - optdef->enum_labels.begin(), newSVpvn_utf8(it->c_str(), it->length(), true));
|
|
(void)hv_stores( hv, "labels", newRV_noinc((SV*)av) );
|
|
}
|
|
|
|
if (optdef->default_value)
|
|
(void)hv_stores( hv, "default", ConfigOption_to_SV(*optdef->default_value.get(), *optdef) );
|
|
(void)hv_store( options_hv, opt_key.c_str(), opt_key.length(), newRV_noinc((SV*)hv), 0 );
|
|
}
|
|
|
|
RETVAL = newRV_noinc((SV*)options_hv);
|
|
OUTPUT:
|
|
RETVAL
|
|
%}
|