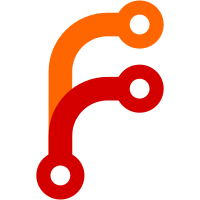
Removed GCode.pm Removed the Perl bindigns for AvoidCrossingPerimeters, OozePrevention, SpiralVase, Wipe Changed the std::set of extruder IDs to vector of IDs. Removed some MSVC compiler warnings, removed obnoxious compiler warnings when compiling the Perl bindings.
58 lines
1.8 KiB
Plaintext
58 lines
1.8 KiB
Plaintext
%module{Slic3r::XS};
|
|
|
|
%{
|
|
#include <xsinit.h>
|
|
#include "libslic3r/GCode.hpp"
|
|
#include "libslic3r/GCode/CoolingBuffer.hpp"
|
|
%}
|
|
|
|
%name{Slic3r::GCode::CoolingBuffer} class CoolingBuffer {
|
|
CoolingBuffer(GCode* gcode)
|
|
%code{% RETVAL = new CoolingBuffer(*gcode); %};
|
|
~CoolingBuffer();
|
|
Ref<GCode> gcodegen();
|
|
|
|
std::string append(std::string gcode, size_t object_id, size_t layer_id, bool support_layer);
|
|
std::string flush();
|
|
};
|
|
|
|
%name{Slic3r::GCode} class GCode {
|
|
GCode();
|
|
~GCode();
|
|
std::string do_export(Print *print, const char *path)
|
|
%code{%
|
|
FILE *file = fopen(path, "wb");
|
|
if (file == nullptr) {
|
|
RETVAL = std::string("Failed to open ") + path + " for writing.";
|
|
} else {
|
|
THIS->do_export(file, *print);
|
|
fclose(file);
|
|
RETVAL = std::string();
|
|
}
|
|
%};
|
|
|
|
Ref<Pointf> origin()
|
|
%code{% RETVAL = &(THIS->origin()); %};
|
|
void set_origin(Pointf* pointf)
|
|
%code{% THIS->set_origin(*pointf); %};
|
|
Ref<Point> last_pos()
|
|
%code{% RETVAL = &(THIS->last_pos()); %};
|
|
|
|
unsigned int layer_count() const;
|
|
void set_layer_count(unsigned int value);
|
|
float elapsed_time() const;
|
|
void set_elapsed_time(float value);
|
|
|
|
void apply_print_config(StaticPrintConfig* print_config)
|
|
%code{%
|
|
if (const PrintConfig* config = dynamic_cast<PrintConfig*>(print_config)) {
|
|
THIS->apply_print_config(*config);
|
|
} else {
|
|
CONFESS("A PrintConfig object was not supplied to apply_print_config()");
|
|
}
|
|
%};
|
|
|
|
Ref<StaticPrintConfig> config()
|
|
%code{% RETVAL = const_cast<StaticPrintConfig*>(dynamic_cast<const StaticPrintConfig*>(&THIS->config())); %};
|
|
};
|