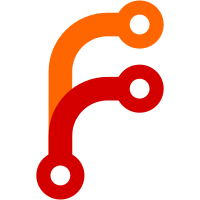
config bundles, project files (3MFs, AMFs). When loading these files, the caller may decide whether to substitute some of the configuration values the current PrusaSlicer version does not understand with some reasonable default value, and whether to report it. If substitution is disabled, an exception is being thrown as before this commit. If substitution is enabled, list of substitutions is returned by the API to be presented to the user. This allows us to introduce for example new firmware flavor key in PrusaSlicer 2.4 while letting PrusaSlicer 2.3.2 to fall back to some default and to report it to the user. When slicing from command line, substutions are performed by default and reported into the console, however substitutions may be either disabled or made silent with the new "config-compatibility" command line option. Substitute enums and bools only. Allow booleans to be parsed as true: "1", "enabled", "on" case insensitive false: "0", "disabled", "off" case insensitive This will allow us in the future for example to switch the draft_shield boolean to an enum with the following values: "disabled" / "enabled" / "limited". Added "enum_bitmask.hpp" - support for type safe sets of options. See for example PresetBundle::load_configbundle(... LoadConfigBundleAttributes flags) for an example of intended usage. WIP: GUI for reporting the list of config substitutions needs to be implemented by @YuSanka.
129 lines
5.0 KiB
C++
129 lines
5.0 KiB
C++
#include <catch2/catch.hpp>
|
|
|
|
#include "libslic3r/libslic3r.h"
|
|
#include "libslic3r/Print.hpp"
|
|
#include "libslic3r/Layer.hpp"
|
|
|
|
#include "test_data.hpp"
|
|
|
|
using namespace Slic3r;
|
|
using namespace Slic3r::Test;
|
|
|
|
SCENARIO("PrintObject: Perimeter generation", "[PrintObject]") {
|
|
GIVEN("20mm cube and default config") {
|
|
WHEN("make_perimeters() is called") {
|
|
Slic3r::Print print;
|
|
Slic3r::Test::init_and_process_print({TestMesh::cube_20x20x20}, print, { { "fill_density", 0 } });
|
|
const PrintObject &object = *print.objects().front();
|
|
THEN("67 layers exist in the model") {
|
|
REQUIRE(object.layers().size() == 66);
|
|
}
|
|
THEN("Every layer in region 0 has 1 island of perimeters") {
|
|
for (const Layer *layer : object.layers())
|
|
REQUIRE(layer->regions().front()->perimeters.entities.size() == 1);
|
|
}
|
|
THEN("Every layer in region 0 has 3 paths in its perimeters list.") {
|
|
for (const Layer *layer : object.layers())
|
|
REQUIRE(layer->regions().front()->perimeters.items_count() == 3);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
SCENARIO("Print: Skirt generation", "[Print]") {
|
|
GIVEN("20mm cube and default config") {
|
|
WHEN("Skirts is set to 2 loops") {
|
|
Slic3r::Print print;
|
|
Slic3r::Test::init_and_process_print({TestMesh::cube_20x20x20}, print, {
|
|
{ "skirt_height", 1 },
|
|
{ "skirt_distance", 1 },
|
|
{ "skirts", 2 }
|
|
});
|
|
THEN("Skirt Extrusion collection has 2 loops in it") {
|
|
REQUIRE(print.skirt().items_count() == 2);
|
|
REQUIRE(print.skirt().flatten().entities.size() == 2);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
SCENARIO("Print: Changing number of solid surfaces does not cause all surfaces to become internal.", "[Print]") {
|
|
GIVEN("sliced 20mm cube and config with top_solid_surfaces = 2 and bottom_solid_surfaces = 1") {
|
|
Slic3r::DynamicPrintConfig config = Slic3r::DynamicPrintConfig::full_print_config();
|
|
config.set_deserialize_strict({
|
|
{ "top_solid_layers", 2 },
|
|
{ "bottom_solid_layers", 1 },
|
|
{ "layer_height", 0.25 }, // get a known number of layers
|
|
{ "first_layer_height", 0.25 }
|
|
});
|
|
Slic3r::Print print;
|
|
Slic3r::Model model;
|
|
Slic3r::Test::init_print({TestMesh::cube_20x20x20}, print, model, config);
|
|
// Precondition: Ensure that the model has 2 solid top layers (39, 38)
|
|
// and one solid bottom layer (0).
|
|
auto test_is_solid_infill = [&print](size_t obj_id, size_t layer_id) {
|
|
const Layer &layer = *(print.objects().at(obj_id)->get_layer((int)layer_id));
|
|
// iterate over all of the regions in the layer
|
|
for (const LayerRegion *region : layer.regions()) {
|
|
// for each region, iterate over the fill surfaces
|
|
for (const Surface &surface : region->fill_surfaces.surfaces)
|
|
CHECK(surface.is_solid());
|
|
}
|
|
};
|
|
print.process();
|
|
test_is_solid_infill(0, 0); // should be solid
|
|
test_is_solid_infill(0, 79); // should be solid
|
|
test_is_solid_infill(0, 78); // should be solid
|
|
WHEN("Model is re-sliced with top_solid_layers == 3") {
|
|
config.set("top_solid_layers", 3);
|
|
print.apply(model, config);
|
|
print.process();
|
|
THEN("Print object does not have 0 solid bottom layers.") {
|
|
test_is_solid_infill(0, 0);
|
|
}
|
|
AND_THEN("Print object has 3 top solid layers") {
|
|
test_is_solid_infill(0, 79);
|
|
test_is_solid_infill(0, 78);
|
|
test_is_solid_infill(0, 77);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
SCENARIO("Print: Brim generation", "[Print]") {
|
|
GIVEN("20mm cube and default config, 1mm first layer width") {
|
|
WHEN("Brim is set to 3mm") {
|
|
Slic3r::Print print;
|
|
Slic3r::Test::init_and_process_print({TestMesh::cube_20x20x20}, print, {
|
|
{ "first_layer_extrusion_width", 1 },
|
|
{ "brim_width", 3 }
|
|
});
|
|
THEN("Brim Extrusion collection has 3 loops in it") {
|
|
REQUIRE(print.brim().items_count() == 3);
|
|
}
|
|
}
|
|
WHEN("Brim is set to 6mm") {
|
|
Slic3r::Print print;
|
|
Slic3r::Test::init_and_process_print({TestMesh::cube_20x20x20}, print, {
|
|
{ "first_layer_extrusion_width", 1 },
|
|
{ "brim_width", 6 }
|
|
});
|
|
THEN("Brim Extrusion collection has 6 loops in it") {
|
|
REQUIRE(print.brim().items_count() == 6);
|
|
}
|
|
}
|
|
WHEN("Brim is set to 6mm, extrusion width 0.5mm") {
|
|
Slic3r::Print print;
|
|
Slic3r::Test::init_and_process_print({TestMesh::cube_20x20x20}, print, {
|
|
{ "first_layer_extrusion_width", 1 },
|
|
{ "brim_width", 6 },
|
|
{ "first_layer_extrusion_width", 0.5 }
|
|
});
|
|
print.process();
|
|
THEN("Brim Extrusion collection has 12 loops in it") {
|
|
REQUIRE(print.brim().items_count() == 14);
|
|
}
|
|
}
|
|
}
|
|
}
|