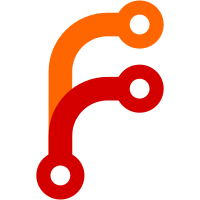
croak() expects printf-style format strings. Calling croak(e.what()) directly causes compilations to fail with -Werror=format-security
86 lines
3.0 KiB
Plaintext
86 lines
3.0 KiB
Plaintext
%module{Slic3r::XS};
|
|
|
|
%{
|
|
#include <xsinit.h>
|
|
#include "libslic3r/GCode.hpp"
|
|
#include "libslic3r/GCode/CoolingBuffer.hpp"
|
|
#include "libslic3r/GCode/PreviewData.hpp"
|
|
%}
|
|
|
|
%name{Slic3r::GCode::CoolingBuffer} class CoolingBuffer {
|
|
CoolingBuffer(GCode* gcode)
|
|
%code{% RETVAL = new CoolingBuffer(*gcode); %};
|
|
~CoolingBuffer();
|
|
Ref<GCode> gcodegen();
|
|
std::string process_layer(std::string gcode, size_t layer_id);
|
|
};
|
|
|
|
%name{Slic3r::GCode} class GCode {
|
|
GCode();
|
|
~GCode();
|
|
void do_export(Print *print, const char *path)
|
|
%code%{
|
|
try {
|
|
THIS->do_export(print, path);
|
|
} catch (std::exception& e) {
|
|
croak("%s\n", e.what());
|
|
}
|
|
%};
|
|
void do_export_w_preview(Print *print, const char *path, GCodePreviewData *preview_data)
|
|
%code%{
|
|
try {
|
|
THIS->do_export(print, path, preview_data);
|
|
} catch (std::exception& e) {
|
|
croak(e.what());
|
|
}
|
|
%};
|
|
|
|
Ref<Pointf> origin()
|
|
%code{% RETVAL = &(THIS->origin()); %};
|
|
void set_origin(Pointf* pointf)
|
|
%code{% THIS->set_origin(*pointf); %};
|
|
Ref<Point> last_pos()
|
|
%code{% RETVAL = &(THIS->last_pos()); %};
|
|
|
|
unsigned int layer_count() const;
|
|
void set_layer_count(unsigned int value);
|
|
void set_extruders(std::vector<unsigned int> extruders)
|
|
%code{% THIS->writer().set_extruders(extruders); THIS->writer().set_extruder(0); %};
|
|
|
|
void apply_print_config(StaticPrintConfig* print_config)
|
|
%code{%
|
|
if (const PrintConfig* config = dynamic_cast<PrintConfig*>(print_config)) {
|
|
THIS->apply_print_config(*config);
|
|
} else {
|
|
CONFESS("A PrintConfig object was not supplied to apply_print_config()");
|
|
}
|
|
%};
|
|
|
|
Ref<StaticPrintConfig> config()
|
|
%code{% RETVAL = const_cast<StaticPrintConfig*>(static_cast<const StaticPrintConfig*>(static_cast<const PrintObjectConfig*>(&THIS->config()))); %};
|
|
};
|
|
|
|
%name{Slic3r::GCode::PreviewData} class GCodePreviewData {
|
|
GCodePreviewData();
|
|
~GCodePreviewData();
|
|
void reset();
|
|
bool empty() const;
|
|
void set_type(int type)
|
|
%code%{
|
|
if ((0 <= type) && (type < GCodePreviewData::Extrusion::Num_View_Types))
|
|
THIS->extrusion.view_type = (GCodePreviewData::Extrusion::EViewType)type;
|
|
%};
|
|
int type() %code%{ RETVAL = (int)THIS->extrusion.view_type; %};
|
|
void set_extrusion_flags(int flags)
|
|
%code%{ THIS->extrusion.role_flags = (unsigned int)flags; %};
|
|
void set_travel_visible(bool visible)
|
|
%code%{ THIS->travel.is_visible = visible; %};
|
|
void set_retractions_visible(bool visible)
|
|
%code%{ THIS->retraction.is_visible = visible; %};
|
|
void set_unretractions_visible(bool visible)
|
|
%code%{ THIS->unretraction.is_visible = visible; %};
|
|
void set_shells_visible(bool visible)
|
|
%code%{ THIS->shell.is_visible = visible; %};
|
|
void set_extrusion_paths_colors(std::vector<std::string> colors);
|
|
};
|