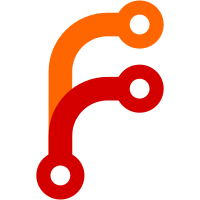
"Multimaterial printer switches filament at the wrong time during a print" https://github.com/prusa3d/Slic3r/issues/607 There was a single layer between the raft top and the object first layer missing on the wipe tower, and after this missing layer all the tool changes were shifted by one layer, meaning two color print had the colors switched.
123 lines
4.1 KiB
Plaintext
123 lines
4.1 KiB
Plaintext
%module{Slic3r::XS};
|
|
|
|
%{
|
|
#include <xsinit.h>
|
|
#include "libslic3r/Layer.hpp"
|
|
%}
|
|
|
|
%name{Slic3r::Layer::Region} class LayerRegion {
|
|
// owned by Layer, no constructor/destructor
|
|
|
|
Ref<Layer> layer();
|
|
Ref<PrintRegion> region();
|
|
|
|
Ref<SurfaceCollection> slices()
|
|
%code%{ RETVAL = &THIS->slices; %};
|
|
Ref<ExtrusionEntityCollection> thin_fills()
|
|
%code%{ RETVAL = &THIS->thin_fills; %};
|
|
Ref<SurfaceCollection> fill_surfaces()
|
|
%code%{ RETVAL = &THIS->fill_surfaces; %};
|
|
Ref<SurfaceCollection> perimeter_surfaces()
|
|
%code%{ RETVAL = &THIS->perimeter_surfaces; %};
|
|
Polygons bridged()
|
|
%code%{ RETVAL = THIS->bridged; %};
|
|
Ref<PolylineCollection> unsupported_bridge_edges()
|
|
%code%{ RETVAL = &THIS->unsupported_bridge_edges; %};
|
|
Ref<ExtrusionEntityCollection> perimeters()
|
|
%code%{ RETVAL = &THIS->perimeters; %};
|
|
Ref<ExtrusionEntityCollection> fills()
|
|
%code%{ RETVAL = &THIS->fills; %};
|
|
|
|
Clone<Flow> flow(FlowRole role, bool bridge = false, double width = -1)
|
|
%code%{ RETVAL = THIS->flow(role, bridge, width); %};
|
|
void prepare_fill_surfaces();
|
|
void make_perimeters(SurfaceCollection* slices, SurfaceCollection* fill_surfaces)
|
|
%code%{ THIS->make_perimeters(*slices, fill_surfaces); %};
|
|
double infill_area_threshold();
|
|
|
|
void export_region_slices_to_svg(const char *path) const;
|
|
void export_region_fill_surfaces_to_svg(const char *path) const;
|
|
void export_region_slices_to_svg_debug(const char *name) const;
|
|
void export_region_fill_surfaces_to_svg_debug(const char *name) const;
|
|
};
|
|
|
|
%name{Slic3r::Layer} class Layer {
|
|
// owned by PrintObject, no constructor/destructor
|
|
|
|
Ref<Layer> as_layer()
|
|
%code%{ RETVAL = THIS; %};
|
|
|
|
int id();
|
|
void set_id(int id);
|
|
Ref<PrintObject> object();
|
|
bool slicing_errors()
|
|
%code%{ RETVAL = THIS->slicing_errors; %};
|
|
coordf_t slice_z()
|
|
%code%{ RETVAL = THIS->slice_z; %};
|
|
coordf_t print_z()
|
|
%code%{ RETVAL = THIS->print_z; %};
|
|
coordf_t height()
|
|
%code%{ RETVAL = THIS->height; %};
|
|
|
|
size_t region_count();
|
|
Ref<LayerRegion> get_region(int idx);
|
|
Ref<LayerRegion> add_region(PrintRegion* print_region);
|
|
|
|
Ref<ExPolygonCollection> slices()
|
|
%code%{ RETVAL = &THIS->slices; %};
|
|
|
|
int ptr()
|
|
%code%{ RETVAL = (int)(intptr_t)THIS; %};
|
|
|
|
Ref<SupportLayer> as_support_layer()
|
|
%code%{ RETVAL = dynamic_cast<SupportLayer*>(THIS); %};
|
|
|
|
void make_slices();
|
|
void merge_slices();
|
|
void make_perimeters();
|
|
void make_fills();
|
|
|
|
void export_region_slices_to_svg(const char *path);
|
|
void export_region_fill_surfaces_to_svg(const char *path);
|
|
void export_region_slices_to_svg_debug(const char *name);
|
|
void export_region_fill_surfaces_to_svg_debug(const char *name);
|
|
};
|
|
|
|
%name{Slic3r::Layer::Support} class SupportLayer {
|
|
// owned by PrintObject, no constructor/destructor
|
|
|
|
Ref<Layer> as_layer()
|
|
%code%{ RETVAL = THIS; %};
|
|
|
|
Ref<ExPolygonCollection> support_islands()
|
|
%code%{ RETVAL = &THIS->support_islands; %};
|
|
Ref<ExtrusionEntityCollection> support_fills()
|
|
%code%{ RETVAL = &THIS->support_fills; %};
|
|
|
|
// copies of some Layer methods, because the parameter wrapper code
|
|
// gets confused about getting a Layer::Support instead of a Layer
|
|
int id();
|
|
void set_id(int id);
|
|
Ref<PrintObject> object();
|
|
bool slicing_errors()
|
|
%code%{ RETVAL = THIS->slicing_errors; %};
|
|
coordf_t slice_z()
|
|
%code%{ RETVAL = THIS->slice_z; %};
|
|
coordf_t print_z()
|
|
%code%{ RETVAL = THIS->print_z; %};
|
|
coordf_t height()
|
|
%code%{ RETVAL = THIS->height; %};
|
|
|
|
size_t region_count();
|
|
Ref<LayerRegion> get_region(int idx);
|
|
Ref<LayerRegion> add_region(PrintRegion* print_region);
|
|
|
|
Ref<ExPolygonCollection> slices()
|
|
%code%{ RETVAL = &THIS->slices; %};
|
|
|
|
void export_region_slices_to_svg(const char *path);
|
|
void export_region_fill_surfaces_to_svg(const char *path);
|
|
void export_region_slices_to_svg_debug(const char *name);
|
|
void export_region_fill_surfaces_to_svg_debug(const char *name);
|
|
};
|