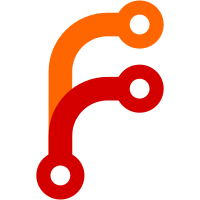
Reworked the FFF testing framework & ConfigBase::set_deserialize() for more compact tests: set_deserialize() now accepts list of key / value pairs. Fixed an incorrect assert in LayerRegion.
271 lines
13 KiB
C++
271 lines
13 KiB
C++
#include <catch2/catch.hpp>
|
|
|
|
#include "libslic3r/libslic3r.h"
|
|
#include "libslic3r/GCodeReader.hpp"
|
|
|
|
#include "test_data.hpp"
|
|
|
|
#include <algorithm>
|
|
#include <regex>
|
|
|
|
using namespace Slic3r;
|
|
using namespace Slic3r::Test;
|
|
|
|
std::regex perimeters_regex("G1 X[-0-9.]* Y[-0-9.]* E[-0-9.]* ; perimeter");
|
|
std::regex infill_regex("G1 X[-0-9.]* Y[-0-9.]* E[-0-9.]* ; infill");
|
|
std::regex skirt_regex("G1 X[-0-9.]* Y[-0-9.]* E[-0-9.]* ; skirt");
|
|
|
|
SCENARIO( "PrintGCode basic functionality", "[PrintGCode]") {
|
|
GIVEN("A default configuration and a print test object") {
|
|
WHEN("the output is executed with no support material") {
|
|
Slic3r::Print print;
|
|
Slic3r::Model model;
|
|
Slic3r::Test::init_print({TestMesh::cube_20x20x20}, print, model, {
|
|
{ "layer_height", "0.2" },
|
|
{ "first_layer_height", "0.2" },
|
|
{ "first_layer_extrusion_width", "0" },
|
|
{ "gcode_comments", "1" },
|
|
{ "start_gcode", "" }
|
|
});
|
|
std::string gcode = Slic3r::Test::gcode(print);
|
|
THEN("Some text output is generated.") {
|
|
REQUIRE(gcode.size() > 0);
|
|
}
|
|
THEN("Exported text contains slic3r version") {
|
|
REQUIRE(gcode.find(SLIC3R_VERSION) != std::string::npos);
|
|
}
|
|
//THEN("Exported text contains git commit id") {
|
|
// REQUIRE(gcode.find("; Git Commit") != std::string::npos);
|
|
// REQUIRE(gcode.find(SLIC3R_BUILD_ID) != std::string::npos);
|
|
//}
|
|
THEN("Exported text contains extrusion statistics.") {
|
|
REQUIRE(gcode.find("; external perimeters extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; perimeters extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; solid infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; top infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; support material extrusion width") == std::string::npos);
|
|
REQUIRE(gcode.find("; first layer extrusion width") == std::string::npos);
|
|
}
|
|
THEN("Exported text does not contain cooling markers (they were consumed)") {
|
|
REQUIRE(gcode.find(";_EXTRUDE_SET_SPEED") == std::string::npos);
|
|
}
|
|
|
|
THEN("GCode preamble is emitted.") {
|
|
REQUIRE(gcode.find("G21 ; set units to millimeters") != std::string::npos);
|
|
}
|
|
|
|
THEN("Config options emitted for print config, default region config, default object config") {
|
|
REQUIRE(gcode.find("; first_layer_temperature") != std::string::npos);
|
|
REQUIRE(gcode.find("; layer_height") != std::string::npos);
|
|
REQUIRE(gcode.find("; fill_density") != std::string::npos);
|
|
}
|
|
THEN("Infill is emitted.") {
|
|
std::smatch has_match;
|
|
REQUIRE(std::regex_search(gcode, has_match, infill_regex));
|
|
}
|
|
THEN("Perimeters are emitted.") {
|
|
std::smatch has_match;
|
|
REQUIRE(std::regex_search(gcode, has_match, perimeters_regex));
|
|
}
|
|
THEN("Skirt is emitted.") {
|
|
std::smatch has_match;
|
|
REQUIRE(std::regex_search(gcode, has_match, skirt_regex));
|
|
}
|
|
THEN("final Z height is 20mm") {
|
|
double final_z = 0.0;
|
|
GCodeReader reader;
|
|
reader.apply_config(print.config());
|
|
reader.parse_buffer(gcode, [&final_z] (GCodeReader& self, const GCodeReader::GCodeLine& line) {
|
|
final_z = std::max<double>(final_z, static_cast<double>(self.z())); // record the highest Z point we reach
|
|
});
|
|
REQUIRE(final_z == Approx(20.));
|
|
}
|
|
}
|
|
WHEN("output is executed with complete objects and two differently-sized meshes") {
|
|
Slic3r::Print print;
|
|
Slic3r::Model model;
|
|
Slic3r::Test::init_print({TestMesh::cube_20x20x20,TestMesh::cube_20x20x20}, print, model, {
|
|
{ "first_layer_extrusion_width", "0" },
|
|
{ "first_layer_height", "0.3" },
|
|
{ "layer_height", "0.2" },
|
|
{ "support_material", "0" },
|
|
{ "raft_layers", "0" },
|
|
{ "complete_objects", "1" },
|
|
{ "gcode_comments", "1" },
|
|
{ "between_objects_gcode", "; between-object-gcode" }
|
|
});
|
|
std::string gcode = Slic3r::Test::gcode(print);
|
|
THEN("Some text output is generated.") {
|
|
REQUIRE(gcode.size() > 0);
|
|
}
|
|
THEN("Infill is emitted.") {
|
|
std::smatch has_match;
|
|
REQUIRE(std::regex_search(gcode, has_match, infill_regex));
|
|
}
|
|
THEN("Perimeters are emitted.") {
|
|
std::smatch has_match;
|
|
REQUIRE(std::regex_search(gcode, has_match, perimeters_regex));
|
|
}
|
|
THEN("Skirt is emitted.") {
|
|
std::smatch has_match;
|
|
REQUIRE(std::regex_search(gcode, has_match, skirt_regex));
|
|
}
|
|
THEN("Between-object-gcode is emitted.") {
|
|
REQUIRE(gcode.find("; between-object-gcode") != std::string::npos);
|
|
}
|
|
THEN("final Z height is 20.1mm") {
|
|
double final_z = 0.0;
|
|
GCodeReader reader;
|
|
reader.apply_config(print.config());
|
|
reader.parse_buffer(gcode, [&final_z] (GCodeReader& self, const GCodeReader::GCodeLine& line) {
|
|
final_z = std::max(final_z, static_cast<double>(self.z())); // record the highest Z point we reach
|
|
});
|
|
REQUIRE(final_z == Approx(20.1));
|
|
}
|
|
THEN("Z height resets on object change") {
|
|
double final_z = 0.0;
|
|
bool reset = false;
|
|
GCodeReader reader;
|
|
reader.apply_config(print.config());
|
|
reader.parse_buffer(gcode, [&final_z, &reset] (GCodeReader& self, const GCodeReader::GCodeLine& line) {
|
|
if (final_z > 0 && std::abs(self.z() - 0.3) < 0.01 ) { // saw higher Z before this, now it's lower
|
|
reset = true;
|
|
} else {
|
|
final_z = std::max(final_z, static_cast<double>(self.z())); // record the highest Z point we reach
|
|
}
|
|
});
|
|
REQUIRE(reset == true);
|
|
}
|
|
THEN("Shorter object is printed before taller object.") {
|
|
double final_z = 0.0;
|
|
bool reset = false;
|
|
GCodeReader reader;
|
|
reader.apply_config(print.config());
|
|
reader.parse_buffer(gcode, [&final_z, &reset] (GCodeReader& self, const GCodeReader::GCodeLine& line) {
|
|
if (final_z > 0 && std::abs(self.z() - 0.3) < 0.01 ) {
|
|
reset = (final_z > 20.0);
|
|
} else {
|
|
final_z = std::max(final_z, static_cast<double>(self.z())); // record the highest Z point we reach
|
|
}
|
|
});
|
|
REQUIRE(reset == true);
|
|
}
|
|
}
|
|
WHEN("the output is executed with support material") {
|
|
std::string gcode = ::Test::slice({TestMesh::cube_20x20x20}, {
|
|
{ "first_layer_extrusion_width", "0" },
|
|
{ "support_material", "1" },
|
|
{ "raft_layers", "3" },
|
|
{ "gcode_comments", "1" }
|
|
});
|
|
THEN("Some text output is generated.") {
|
|
REQUIRE(gcode.size() > 0);
|
|
}
|
|
THEN("Exported text contains extrusion statistics.") {
|
|
REQUIRE(gcode.find("; external perimeters extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; perimeters extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; solid infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; top infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; support material extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; first layer extrusion width") == std::string::npos);
|
|
}
|
|
THEN("Raft is emitted.") {
|
|
REQUIRE(gcode.find("; raft") != std::string::npos);
|
|
}
|
|
}
|
|
WHEN("the output is executed with a separate first layer extrusion width") {
|
|
std::string gcode = ::Test::slice({ TestMesh::cube_20x20x20 }, {
|
|
{ "first_layer_extrusion_width", "0.5" }
|
|
});
|
|
THEN("Some text output is generated.") {
|
|
REQUIRE(gcode.size() > 0);
|
|
}
|
|
THEN("Exported text contains extrusion statistics.") {
|
|
REQUIRE(gcode.find("; external perimeters extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; perimeters extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; solid infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; top infill extrusion width") != std::string::npos);
|
|
REQUIRE(gcode.find("; support material extrusion width") == std::string::npos);
|
|
REQUIRE(gcode.find("; first layer extrusion width") != std::string::npos);
|
|
}
|
|
}
|
|
WHEN("Cooling is enabled and the fan is disabled.") {
|
|
std::string gcode = ::Test::slice({ TestMesh::cube_20x20x20 }, {
|
|
{ "cooling", "1" },
|
|
{ "disable_fan_first_layers", "5" }
|
|
});
|
|
THEN("GCode to disable fan is emitted."){
|
|
REQUIRE(gcode.find("M107") != std::string::npos);
|
|
}
|
|
}
|
|
WHEN("end_gcode exists with layer_num and layer_z") {
|
|
std::string gcode = ::Test::slice({ TestMesh::cube_20x20x20 }, {
|
|
{ "end_gcode", "; Layer_num [layer_num]\n; Layer_z [layer_z]" },
|
|
{ "layer_height", "0.1" },
|
|
{ "first_layer_height", "0.1" }
|
|
});
|
|
THEN("layer_num and layer_z are processed in the end gcode") {
|
|
REQUIRE(gcode.find("; Layer_num 199") != std::string::npos);
|
|
REQUIRE(gcode.find("; Layer_z 20") != std::string::npos);
|
|
}
|
|
}
|
|
WHEN("current_extruder exists in start_gcode") {
|
|
{
|
|
std::string gcode = ::Test::slice({ TestMesh::cube_20x20x20 }, {
|
|
{ "start_gcode", "; Extruder [current_extruder]" }
|
|
});
|
|
THEN("current_extruder is processed in the start gcode and set for first extruder") {
|
|
REQUIRE(gcode.find("; Extruder 0") != std::string::npos);
|
|
}
|
|
}
|
|
{
|
|
DynamicPrintConfig config = DynamicPrintConfig::full_print_config();
|
|
config.set_num_extruders(4);
|
|
config.set_deserialize({
|
|
{ "start_gcode", "; Extruder [current_extruder]" },
|
|
{ "infill_extruder", "2" },
|
|
{ "solid_infill_extruder", "2" },
|
|
{ "perimeter_extruder", "2" },
|
|
{ "support_material_extruder", "2" },
|
|
{ "support_material_interface_extruder", "2" }
|
|
});
|
|
std::string gcode = Slic3r::Test::slice({TestMesh::cube_20x20x20}, config);
|
|
THEN("current_extruder is processed in the start gcode and set for second extruder") {
|
|
REQUIRE(gcode.find("; Extruder 1") != std::string::npos);
|
|
}
|
|
}
|
|
}
|
|
|
|
WHEN("layer_num represents the layer's index from z=0") {
|
|
std::string gcode = ::Test::slice({ TestMesh::cube_20x20x20, TestMesh::cube_20x20x20 }, {
|
|
{ "complete_objects", "1" },
|
|
{ "gcode_comments", "1" },
|
|
{ "layer_gcode", ";Layer:[layer_num] ([layer_z] mm)" },
|
|
{ "layer_height", "1.0" },
|
|
{ "first_layer_height", "1.0" }
|
|
});
|
|
// End of the 1st object.
|
|
size_t pos = gcode.find(";Layer:19 ");
|
|
THEN("First and second object last layer is emitted") {
|
|
// First object
|
|
REQUIRE(pos != std::string::npos);
|
|
pos += 10;
|
|
REQUIRE(pos < gcode.size());
|
|
double z = 0;
|
|
REQUIRE((sscanf(gcode.data() + pos, "(%lf mm)", &z) == 1));
|
|
REQUIRE(z == Approx(20.));
|
|
// Second object
|
|
pos = gcode.find(";Layer:39 ", pos);
|
|
REQUIRE(pos != std::string::npos);
|
|
pos += 10;
|
|
REQUIRE(pos < gcode.size());
|
|
REQUIRE((sscanf(gcode.data() + pos, "(%lf mm)", &z) == 1));
|
|
REQUIRE(z == Approx(20.));
|
|
}
|
|
}
|
|
}
|
|
}
|