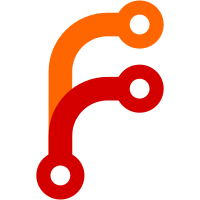
Conflicts: lib/Slic3r.pm lib/Slic3r/ExPolygon.pm lib/Slic3r/Fill.pm lib/Slic3r/Fill/Rectilinear.pm lib/Slic3r/GCode.pm lib/Slic3r/GUI/Plater.pm lib/Slic3r/Geometry/Clipper.pm lib/Slic3r/Layer/Region.pm lib/Slic3r/Print.pm lib/Slic3r/Print/Object.pm lib/Slic3r/TriangleMesh.pm t/shells.t xs/MANIFEST
65 lines
1.6 KiB
Plaintext
65 lines
1.6 KiB
Plaintext
%module{Slic3r::XS};
|
|
|
|
%{
|
|
#include <myinit.h>
|
|
#include "SurfaceCollection.hpp"
|
|
%}
|
|
|
|
%name{Slic3r::Surface::Collection} class SurfaceCollection {
|
|
~SurfaceCollection();
|
|
void clear()
|
|
%code{% THIS->surfaces.clear(); %};
|
|
%{
|
|
|
|
SurfaceCollection*
|
|
SurfaceCollection::new(...)
|
|
CODE:
|
|
RETVAL = new SurfaceCollection;
|
|
// ST(0) is class name, others are surfaces
|
|
RETVAL->surfaces.resize(items-1);
|
|
for (unsigned int i = 1; i < items; i++) {
|
|
// Note: a COPY of the input is stored
|
|
RETVAL->surfaces[i-1] = *(Surface *)SvIV((SV*)SvRV( ST(i) ));
|
|
}
|
|
OUTPUT:
|
|
RETVAL
|
|
|
|
SV*
|
|
SurfaceCollection::arrayref()
|
|
CODE:
|
|
AV* av = newAV();
|
|
av_fill(av, THIS->surfaces.size()-1);
|
|
int i = 0;
|
|
for (Surfaces::iterator it = THIS->surfaces.begin(); it != THIS->surfaces.end(); ++it) {
|
|
SV* sv = newSV(0);
|
|
sv_setref_pv( sv, "Slic3r::Surface", new Surface(*it) );
|
|
av_store(av, i++, sv);
|
|
}
|
|
RETVAL = newRV_noinc((SV*)av);
|
|
OUTPUT:
|
|
RETVAL
|
|
|
|
void
|
|
SurfaceCollection::append(...)
|
|
CODE:
|
|
for (unsigned int i = 1; i < items; i++) {
|
|
THIS->surfaces.push_back(*(Surface *)SvIV((SV*)SvRV( ST(i) )));
|
|
}
|
|
|
|
void
|
|
SurfaceCollection::replace(index, surface)
|
|
int index
|
|
Surface* surface
|
|
CODE:
|
|
THIS->surfaces[index] = *surface;
|
|
|
|
void
|
|
SurfaceCollection::set_surface_type(index, surface_type)
|
|
int index
|
|
SurfaceType surface_type;
|
|
CODE:
|
|
THIS->surfaces[index].surface_type = surface_type;
|
|
|
|
%}
|
|
};
|