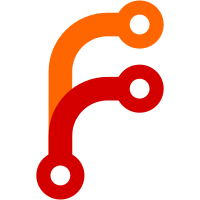
The github module only authenticate by query string, and this method is deprecated: https://developer.github.com/changes/2019-11-05-deprecated-passwords-and-authorizations-api/#authenticating-using-query-parameters There is no reason to remove it before the method stop working, so I've made possible to the user choose which authentication method he will use: * The parameter token remain unchanged. * If the parameter user is passed then the module will use the not deprecated method, passing user and token on the body of the requisition. Otherwise the module will use the deprecated method. Co-authored-by: Lucas <araujo.lucasvale@gmail.com> Fixes #2002
41 lines
1019 B
C++
41 lines
1019 B
C++
#pragma once
|
|
|
|
#include "modules/meta/timer_module.hpp"
|
|
#include "settings.hpp"
|
|
#include "utils/http.hpp"
|
|
|
|
POLYBAR_NS
|
|
|
|
namespace modules {
|
|
/**
|
|
* Module used to query the GitHub API for notification count
|
|
*/
|
|
class github_module : public timer_module<github_module> {
|
|
public:
|
|
explicit github_module(const bar_settings&, string);
|
|
|
|
bool update();
|
|
bool build(builder* builder, const string& tag) const;
|
|
string get_format() const;
|
|
|
|
private:
|
|
void update_label(int);
|
|
int get_number_of_notification();
|
|
string request();
|
|
static constexpr auto TAG_LABEL = "<label>";
|
|
static constexpr auto TAG_LABEL_OFFLINE = "<label-offline>";
|
|
static constexpr auto FORMAT_OFFLINE = "format-offline";
|
|
|
|
label_t m_label{};
|
|
label_t m_label_offline{};
|
|
string m_api_url;
|
|
string m_user;
|
|
string m_accesstoken{};
|
|
unique_ptr<http_downloader> m_http{};
|
|
bool m_empty_notifications{false};
|
|
std::atomic<bool> m_offline{false};
|
|
};
|
|
} // namespace modules
|
|
|
|
POLYBAR_NS_END
|