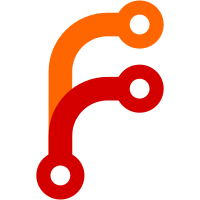
The %{PR} tag is introduced for this. It resets all colors as well as the activation of the underline and overline and font. This has become necessary because we don't track what raw tags a user injects into the formatting string and otherwise their raw tags could bleed through. This doesn't touch action tags because even before raw action tags weren't being tracked. Action tags also have the requirement that they have to be used in pairs, so closing them prematurely could break things (for example with click actions for the entire bar)
84 lines
2.0 KiB
C++
84 lines
2.0 KiB
C++
#pragma once
|
|
|
|
#include <map>
|
|
|
|
#include "common.hpp"
|
|
#include "components/types.hpp"
|
|
|
|
POLYBAR_NS
|
|
|
|
using std::map;
|
|
|
|
// fwd decl
|
|
namespace drawtypes {
|
|
class label;
|
|
using label_t = shared_ptr<label>;
|
|
}
|
|
using namespace drawtypes;
|
|
|
|
class builder {
|
|
public:
|
|
explicit builder(const bar_settings& bar);
|
|
|
|
void reset();
|
|
string flush();
|
|
void append(string text);
|
|
void node(string str, bool add_space = false);
|
|
void node(string str, int font_index, bool add_space = false);
|
|
void node(const label_t& label, bool add_space = false);
|
|
void node_repeat(const string& str, size_t n, bool add_space = false);
|
|
void node_repeat(const label_t& label, size_t n, bool add_space = false);
|
|
void offset(int pixels = 0);
|
|
void space(size_t width);
|
|
void space();
|
|
void remove_trailing_space(size_t len);
|
|
void remove_trailing_space();
|
|
void font(int index);
|
|
void font_close();
|
|
void background(string color);
|
|
void background_close();
|
|
void color(string color);
|
|
void color_alpha(string alpha);
|
|
void color_close();
|
|
void line_color(const string& color);
|
|
void line_color_close();
|
|
void overline_color(string color);
|
|
void overline_color_close();
|
|
void underline_color(string color);
|
|
void underline_color_close();
|
|
void overline(const string& color = "");
|
|
void overline_close();
|
|
void underline(const string& color = "");
|
|
void underline_close();
|
|
void control(controltag tag);
|
|
void cmd(mousebtn index, string action, bool condition = true);
|
|
void cmd(mousebtn index, string action, const label_t& label);
|
|
void cmd_close(bool condition = true);
|
|
|
|
protected:
|
|
string background_hex();
|
|
string foreground_hex();
|
|
|
|
string get_label_text(const label_t& label);
|
|
|
|
void tag_open(syntaxtag tag, const string& value);
|
|
void tag_open(attribute attr);
|
|
void tag_close(syntaxtag tag);
|
|
void tag_close(attribute attr);
|
|
|
|
private:
|
|
const bar_settings m_bar;
|
|
string m_output;
|
|
|
|
map<syntaxtag, int> m_tags{};
|
|
map<syntaxtag, string> m_colors{};
|
|
map<attribute, bool> m_attrs{};
|
|
|
|
int m_fontindex{0};
|
|
|
|
string m_background{};
|
|
string m_foreground{};
|
|
};
|
|
|
|
POLYBAR_NS_END
|