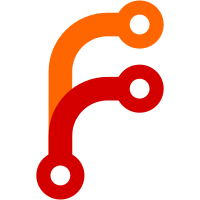
Replaced eval { die } construct with a bool return value indicating success or failure of an automatic arrangement of parts on the print bed. Don't know exactly what is happening here, but throwing a "die" inside a XS function and then catching it inside an eval {} block is suspcious.
66 lines
2.4 KiB
C++
66 lines
2.4 KiB
C++
#ifndef slic3r_Geometry_hpp_
|
|
#define slic3r_Geometry_hpp_
|
|
|
|
#include "libslic3r.h"
|
|
#include "BoundingBox.hpp"
|
|
#include "ExPolygon.hpp"
|
|
#include "Polygon.hpp"
|
|
#include "Polyline.hpp"
|
|
|
|
#include "boost/polygon/voronoi.hpp"
|
|
using boost::polygon::voronoi_builder;
|
|
using boost::polygon::voronoi_diagram;
|
|
|
|
namespace Slic3r { namespace Geometry {
|
|
|
|
Polygon convex_hull(Points points);
|
|
Polygon convex_hull(const Polygons &polygons);
|
|
void chained_path(const Points &points, std::vector<Points::size_type> &retval, Point start_near);
|
|
void chained_path(const Points &points, std::vector<Points::size_type> &retval);
|
|
template<class T> void chained_path_items(Points &points, T &items, T &retval);
|
|
bool directions_parallel(double angle1, double angle2, double max_diff = 0);
|
|
template<class T> bool contains(const std::vector<T> &vector, const Point &point);
|
|
double rad2deg(double angle);
|
|
double rad2deg_dir(double angle);
|
|
double deg2rad(double angle);
|
|
void simplify_polygons(const Polygons &polygons, double tolerance, Polygons* retval);
|
|
|
|
double linint(double value, double oldmin, double oldmax, double newmin, double newmax);
|
|
bool arrange(
|
|
// input
|
|
size_t num_parts, const Pointf &part_size, coordf_t gap, const BoundingBoxf* bed_bounding_box,
|
|
// output
|
|
Pointfs &positions);
|
|
|
|
class MedialAxis {
|
|
public:
|
|
Lines lines;
|
|
const ExPolygon* expolygon;
|
|
double max_width;
|
|
double min_width;
|
|
MedialAxis(double _max_width, double _min_width, const ExPolygon* _expolygon = NULL)
|
|
: max_width(_max_width), min_width(_min_width), expolygon(_expolygon) {};
|
|
void build(ThickPolylines* polylines);
|
|
void build(Polylines* polylines);
|
|
|
|
private:
|
|
class VD : public voronoi_diagram<double> {
|
|
public:
|
|
typedef double coord_type;
|
|
typedef boost::polygon::point_data<coordinate_type> point_type;
|
|
typedef boost::polygon::segment_data<coordinate_type> segment_type;
|
|
typedef boost::polygon::rectangle_data<coordinate_type> rect_type;
|
|
};
|
|
VD vd;
|
|
std::set<const VD::edge_type*> edges, valid_edges;
|
|
std::map<const VD::edge_type*, std::pair<coordf_t,coordf_t> > thickness;
|
|
void process_edge_neighbors(const VD::edge_type* edge, ThickPolyline* polyline);
|
|
bool validate_edge(const VD::edge_type* edge);
|
|
const Line& retrieve_segment(const VD::cell_type* cell) const;
|
|
const Point& retrieve_endpoint(const VD::cell_type* cell) const;
|
|
};
|
|
|
|
} }
|
|
|
|
#endif
|